Introduction
As you may already know, React is a JavaScript library for building user interfaces. React constructs these interfaces using components, which is where the magic happens. Components are reusable, meaning you can use them in multiple places simultaneously. In short, React makes front-end code more reusable and efficient.
React was developed by the Facebook team, and Facebook itself is built on React, proving its power with millions of users. React is commonly used for developing single-page and mobile applications.
However, the focus of this article isn’t on React itself but on React Hooks, introduced in React version 16.8. Hooks are special JavaScript functions that enable additional functionality within React components. Although understanding React class components is helpful for fully grasping Hooks, it’s not a strict requirement. This article will introduce React Hooks and serve as a starting point for a series on this topic.
Hooks in React are used exclusively within functional components and cannot be used inside class components. This brings up an important question: if you’re learning React now, should you start with functional components and Hooks, or with class components? What’s the correct approach?
The answer isn’t simple. React is increasingly focused on functional components with Hooks, and this approach is likely to be the future standard. However, understanding class components is beneficial, especially when working on established projects where class components may still be in use. React has committed to supporting class components in future versions, and both component types can coexist. There’s a wealth of resources for learning React online; I personally recommend this one:
Most Used React Hooks
As I mentioned earlier, React Hooks are built-in functions that enable various functionalities. Although there are 15 Hooks, here are seven of the most important ones:
useState
useEffect
useContext
useReducer
useCallback
useMemo
useRef
I will introduce these Hooks in a series of posts, starting with the most commonly used one, useState
.
Rules of React Hooks
There are a few essential rules for using Hooks:
- Only call Hooks at the top level of a React component.
- Do not call Hooks inside loops, conditions, or nested functions.
- Only call Hooks within React components; they cannot be used in regular JavaScript functions.
useState hook
Let’s look at how the useState
Hook works with a simple variable.
This Hook re-renders a component whenever the value of a variable changes. Below is a React component, Counter
, which has two counters: one implemented with the useState
Hook and one without it.
import React, { useState } from 'react';
const Counter = () => {
const [x, setX] = useState(0);
let y = 0;
return (
<div>
<h2>Count X - {x}</h2>
<div><button>Increment X</button></div>
<h2>Count Y - {y}</h2>
<div><button>Increment Y</button></div>
</div>
);
}
export default Counter;
The line const [x, setX] = useState(0)
declares a variable x
with an initial value of 0
and provides a setter function, setX
, to update x
. For comparison, we also declare a variable y
without using useState
.
On the screen it looks like this:
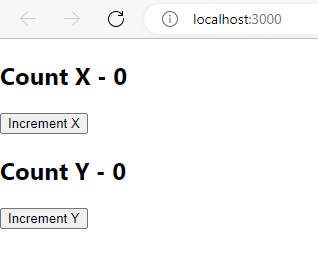
To observe the difference in behavior, let’s add onClick
methods to both buttons:
import React, { useState} from 'react'
const Counter = () => {
const [x, setX] = useState(0)
let y = 0;
const xHandler = ()=> {
setX(x+1)
}
const yHandler = ()=> {
y=y+1
}
return (
<div>
<h2>Count X - {x}</h2>
<div><button onClick={()=> xHandler()}>Increment X</button></div>
<h2>Count Y - {y}</h2>
<div><button onClick={()=>yHandler()}>Increment Y</button></div>
</div>
)
}
export default Counter
Clicking the buttons reveals that changes to x
are visible on the page, while changes to y
are not. This is because x
is a stateful variable, thanks to the useState
Hook, which triggers a re-render on every change, while y
remains stateless.
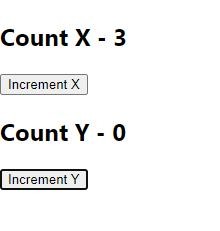
useState hook with object
With React, you can track the state of not only primitive variables like numbers, strings, and booleans but also more complex structures like objects or arrays. Here’s an example:
import React, { useState } from 'react';
const dev1 = {
name: 'Dimche Trifunov',
position: 'developer',
country: 'North Macedonia'
};
const Developer = () => {
const [dev, setDev] = useState(dev1);
return (
<div>
<h3>{dev.name}</h3>
<h3>{dev.position}</h3>
<h3>{dev.country}</h3>
</div>
);
}
export default Developer;
In this Developer
component, the state is a JSON object rather than a primitive variable. The variable dev
has an initial state of dev1
, and there is also a setter function setDev
to update it.
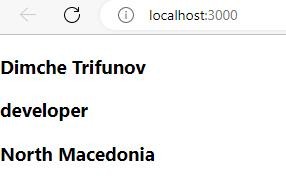
Next, let’s add a button that, when clicked, will change the value of the dev
variable. This will trigger a re-render of the component because dev
is stateful:
import React, { useState } from 'react';
const dev1 = {
name: 'Dimche Trifunov',
position: 'developer',
country: 'North Macedonia'
};
const dev2 = {
name: 'Herbert Straus',
position: 'developer',
country: 'Germany'
};
const Developer = () => {
const [dev, setDev] = useState(dev1);
const devHandler = () => {
setDev(dev2);
};
return (
<div>
<h3>{dev.name}</h3>
<h3>{dev.position}</h3>
<h3>{dev.country}</h3>
<button onClick={devHandler}>Change Developer</button>
</div>
);
}
export default Developer;
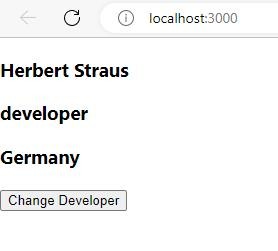
This code demonstrates that when the dev
variable is updated, the component re-renders to reflect the new state.